ASP.NET MVC: Using JavaScript with Ajax and Razor view
https://bitoftech.net/
View
<button id="btnGetData" type="button" class="btn btn-primary"> @Resources.GetData</button>
Controller
The complete list of objects is (they all receive the ControllerContext as the contructor parameter):
- FormValueProvider: search for data in the body (Request.Form)
- RouteDataValueProvider: search for data in the route (RouteData.Value)
- QueryStringValueProvider: search for data in the query string (Request.QueryString)
- HttpFileCollectionValueProvider: search for uploaded files (Request.Files)
System.Web.Mvc.HttpPostAttribute & System.Web.Http.HttpPostAttribute
- System.Web.Mvc.HttpPostAttribute
- System.Web.Http.HttpPostAttribute
IHttpActionResult & HttpResponseMessage
https://docs.microsoft.com/en-us/aspnet/web-api/overview/getting-started-with-aspnet-web-api/action-results
https://www.infoworld.com/article/3192176/my-two-cents-on-using-the-ihttpactionresult-interface-in-webapi.html
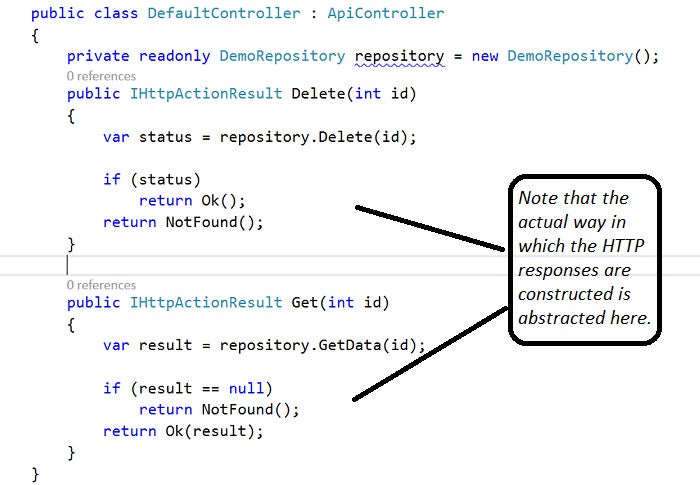
[FromBody]
https://docs.microsoft.com/en-us/aspnet/web-api/overview/formats-and-model-binding/parameter-binding-in-aspnet-web-api#using-frombody
FromBodyAttribute Class (System.Web.Http)
// POST /api/Product/Post
[HttpPost]
public HttpResponseMessage Post([FromBody] Product product)
{
var name = product.Name; // "Product Name"
var price = product.Price; // 0.50
}
Model Binding
https://docs.microsoft.com/en-us/aspnet/web-api/overview/formats-and-model-binding/parameter-binding-in-aspnet-web-api
You can use one of the following attributes to specify the source to use for any given target:
Model
// a complex object
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
JSON
{
"Name" : "Product Name",
"Price" : 0.50
}
Action Get
// GET: /Home/GetData
[HttpGet]
public ActionResult GetData()
{
try
{
string actionName = this.ControllerContext.RouteData.Values["action"].ToString();
string controllerName = this.ControllerContext.RouteData.Values["controller"].ToString();
string redirectUrl = Utilities.GetPageUrl(actionName, controllerName);
string loginUrl = Utilities.GetLoginUrl();
string loginUrlEncrypted = loginUrl;
ViewBag.Url = loginUrl + redirectUrl;
return Json(new { Success = true, Data = loginUrlEncrypted }, JsonRequestBehavior.AllowGet);
}
catch
{
return Json(new { Success = false }, JsonRequestBehavior.AllowGet);
}
}
Action Post
// POST: /Home/SaveData
[HttpPost]
public ActionResult SaveData([FromBody] Product product)
{
try
{
string actionName = this.ControllerContext.RouteData.Values["action"].ToString();
string controllerName = this.ControllerContext.RouteData.Values["controller"].ToString();
bool success = Utilities.SaveProduct(product);
return Json(new { Success = success, Message = "Success" }, JsonRequestBehavior.AllowGet);
}
catch (Exception ex)
{
return Json(new { Success = false, Message = ex.ToString() }, JsonRequestBehavior.AllowGet);
}
}
Call AJAX using JavaScript / jQuery
<script>
window.HomeSaveData = '@Url.Action("SaveData", "Home")';
$(function () {
var obj =
{
"Name" : "Product Name",
"Price" : 0.50
};
debugger;
$.ajax({
url: window.HomeSaveData,
type: "POST",
dataType: "json",
data: JSON.stringify(obj);
contentType: 'application/json; charset=utf-8',
success: function (resultData) {
debugger;
if (resultData.Success = false) {
debugger;
} else {
var message = resultData.Message;
debugger;
}
},
error: function (jqXHR, textStatus, errorThrown) {
},
timeout: 120000
});
});
</script>
Call AJAX using JavaScript / jQuery
<script>
window.HomeGetData = '@Url.Action("GetData", "Home")';
$(function () {
$.ajax({
url: window.HomeGetData,
type: "GET",
dataType: "json",
contentType: 'application/json; charset=utf-8',
success: function (resultData) {
debugger;
if (resultData.Success = false) {
debugger;
} else {
var data = resultData.Data;
debugger;
}
},
error: function (jqXHR, textStatus, errorThrown) {
},
timeout: 120000
});
});
</script>