iCACLS.exe (edit)
https://docs.microsoft.com/en-us/iis/manage/creating-websites/select-a-provisioning-option
https://www.c-sharpcorner.com/search/IIS
List files and their permissions (access) in command line:
iCACLS C:\inetpub\wwwroot\Sites\Site1
How to reset NTFS permissions with iCACLS
iCACLS * /T /Q /C /RESET
iCACLS (link)
c:\windows\system32\icacls
c:\windows\system32\icacls c:\folder /grant "domain\user":(OI)(CI)M
c:\windows\system32\icacls c:\folder /grant "everyone":(OI)(CI)M
c:\windows\system32\icacls c:\folder /grant "Authenticated Users":(OI)(CI)M
icacls systax for recursively adding permissions for Administrators to a folder without altering existing permissions?
icacls "<root folder>" /grant "Domain Admins":F /t
would add Full Access to the "Domain Admins" group to the "root folder" and every folder within.
If you add ":r" after Grant then the permissions would be replaced instead of being added.
icacls "<root folder>" /grant:r "Domain Admins":F /t
The basic permissions are:
Full Control (F)
Modify (M)
Read & Execute (RX)
List Folder Contents (X,RD,RA,REA,RC)
Read (R)
Write (W)
Advanced permissions are:
Full Control (F)
Traverse folder / execute file (X)
List folder / read data (RD)
Read attributes (RA)
Read extended attributes (REA)
Create file / write data (WD)
Create folders / append data (AD)
Write attributes (WA)
Write extended attributes (WEA)
Delete subfolders and files (DC)
Delete (D)
Read permissions (RC)
Change permissions (WDAC)
Take ownership (WO)
You can also specify the inheritance for the folders:
This folder only
This folder, subfolders and files (OI)(CI)
This folder and subfolders (CI)
This folder and files (OI)
Subfolders and files only (OI)(CI)(NP)(IO)
Subfolders only (CI)(IO)
Files only (OI)(IO)
Xcopy.exe
xcopy /s /y "C:\inetpub\wwwroot\Sites\Site1\*.*" "D:\Sites\Site1\"
https://support.microsoft.com/en-us/help/323007/how-to-copy-a-folder-to-another-folder-and-retain-its-permissions
xcopy /S /Q /Y /F "C:\inetpub\wwwroot\Sites\Site1" "D:\Sites\Site1"
xcopy /S /Q /Y /F "C:\inetpub\wwwroot\Sites\Site1" "D:\Sites\Site1"
xcopy /S /I /Q /Y /F "C:\inetpub\wwwroot\Sites\Site1" "D:\Sites\Site2"
xcopy /S /I /Q /Y /F "C:\inetpub\wwwroot\Sites\Site1" "D:\Sites\Site2"
/E - Copies folders and subfolders, including empty ones.
/H - Copies hidden and system files also.
/K - Copies attributes. Typically, Xcopy resets read-only attributes.
/O - Copies file ownership and ACL information.
/X - Copies file audit settings (implies /O).
/X - Copies file audit settings and system access control list (SACL) information (implies /o).
/O - Copies file ownership and discretionary access control list (DACL) information.
/X – Copies file audit settings and file ownership and ACL information.
/H – Copies hidden and system files.
/E – Copies directories and subdirectories, including empty ones.
/V – Verifies each new file.
/D – Copies files changed on or after the specified date (D:m-d-y).If no date is given, copies only those files whose source time is newer than the destination time.
/Y – Suppresses prompting to confirm you want to overwrite an existing destination file.
Windows Server 2016
- Windows Server 2016
- IIS 10 (Windows 10 and Windows Server 2016)
- MS SQL Server 2017
- Office 365 Account
5.0 |
Built-in component of Windows 2000. |
Windows 2000 |
5.1 |
Built-in component of Windows XP Professional. |
Windows XP Professional |
6.0 |
Built-in component of Windows Server 2003. |
WIndows Server 2003 |
7.0 |
Built-in component of Windows Vista and Windows Server 2008. |
Windows Vista and WIndows Server 2008 |
7.5 |
Built-in component of Windows 7 and Windows Server 2008 R2. |
Windows 7 and Windows Server 2008 R2 |
8.0 |
Built-in component of Windows 8 and Windows Server 2012. |
Windows 8 and Windows Server 2012 |
8.5 |
Built-in component of Windows 8.1 and Windows Server 2012 R2. |
Windows 8.1 and Windows Server 2012 R2 |
10.0 |
Built-in component of Windows 10 and Windows Server 2016. |
Windows 10 and Windows Server 2016 |
IIS version?
https://gallery.technet.microsoft.com/how-to-obtain-versions-of-7875ac84
Windows Server 2016 Security Guide
https://download.microsoft.com/download/5/8/5/585DF9E9-D3D6-410A-8B51-81C7FC9A727C/Windows_Server_2016_Security_Guide_EN_US.pdf
Permissions
https://support.microsoft.com/en-us/help/271071/how-to-set-minimum-ntfs-permissions-and-user-rights-for-iis-5-x-or-iis
https://theitbros.com/using-icacls-to-list-folder-permissions-and-manage-files/
AppCmd.exe
http://techgenix.com/configuring-iis-7-command-line-appcmdexe-part1/
Typically, each of these commands would be used with an object type that you are asking AppCmd.exe to perform the requested function on.
Possibilities of the object type are:
- Site – IIS virtual site
- App – IIS application
- Vdir – IIS virtual directory
- Apppool – IIS application pool
- Config – IIS general configuration
- Backup – IIS server configuration backups (and the restore command is also available)
- Wp – IIS worker processes
- Request – active HTTP requests
- Module – IIS server administration modules
- Trace – IIS server trace logs
AppCmd.exe
AppCmd is the built-in CLI tool for configuring and managing IIS. You can use it to create sites and app pools, link virtual directories, and edit configurations. Let’s look at a few things it can do.
First of all, add %systemroot%\system32\inetsrv\ to your path so that you can run appcmd from a command prompt in any location.
Run the command prompt as administrator.
setx PATH "%PATH%;%systemroot%\system32\inetsrv\"
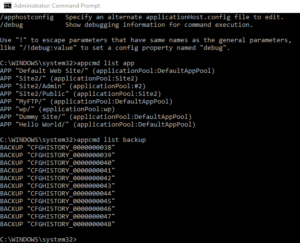
Try the following commands to explore appcmd:
- Run appcmd /? to see the help text
- See what apps are running with appcmd list app
- Use appcmd list backup to see backups of your IIS config
Add site
appcmd add site /name:"Dummy Site" /id:10 /bindings:http/*:81:
Now list apps again. You won’t see the new site you’ve added because it isn’t considered an app. If you go to the GUI and refresh your sites, you’ll see the new site there, but it’ll be broken. We need to add an app using appcmd.
appcmd add app /site.name:"Dummy Site" /path:"/"
This will only add the app to the site at the root. It will create an app named “Dummy Site/”. We still need to link the app to a virtual directory then point that to a physical path.
appcmd add vdir /app.name:"Dummy Site/" path:"/"
appcmd set vdir "Dummy Site/" /physicalPath:"c:\inetpub\wwwroot"
This is the verbose way to use appcmd to create an IIS site. There’s an easier way.
The EZ way
You can save a lot of keystrokes while creating the site if you set the physicalPath parameter in the first command. This will do the whole thing in one shot:
appcmd add site /name:"Dummy Site" /id:10 /bindings:http/*:81: /physicalPath:"c:\inetpub\wwwroot"
But knowing the other commands gives you a better idea of how an IIS app really works under the hood. In the past, I’ve used appcmd in the post-build script of ASP.NET proj files to ensure the site was set up locally on new developer machines. You can also do a backup and restore of IIS config using appcmd.
Deploying updates
To give you one more idea about using appcmd, consider doing the following:
- Create a “sites” folder.
- Create a subfolder for each site.
- Deploy versions to subfolders under each of those.
- Stage new versions.
- Use appcmd to update the site to use the new folder.
Given an app named “Hello World” pointing to C:\Sites\HelloWorld\1.0.0 and a new build “1.0.1” that’s been staged in C:\Sites\HelloWorld\1.0.1 , when it’s time to go live, then you can use the following command to flip the site to the new version:
appcmd set vdir "Dummy Site/" /physicalPath:"c:\Sites\HelloWorld\1.0.1"
appcmd recycle apppool /apppool.name:defaultapppool
And if you need to roll back your site, run the following:
appcmd set vdir "Dummy Site/" /physicalPath:"c:\Sites\HelloWorld\1.0.0"
appcmd recycle apppool /apppool.name:defaultapppool
Here’s a great guide from Microsoft with more information on using AppCmd.
IIS reset
“iisreset” is a separate executable used to stop, start, restart IIS or event to reboot the computer (/REBOOT). You can pass it a “computername” parameter to have it control IIS on another computer. You will need to run this as admin. Many developers and system admins like to use this after a deployment, and that’s not a bad idea either!
References
https://stackify.com/iis-web-server/
iCACLS - Set Permissions for IIS_IUSRS group (IIS7)
icacls "D:\Website\nopcommerce\App_Data" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\bin" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Content" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Content\Images" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Content\Images\Thumbs" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Content\Images\Uploaded" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Content\files\ExportImport" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Plugins" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Plugins\bin" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\Global.asax" /grant IIS_IUSRS:(OI)(CI)F
icacls "D:\Website\nopcommerce\web.config" /grant IIS_IUSRS:(OI)(CI)F