Windows Forms Application (edit)
- Windows Forms Application (WinForms)
- Universal Windows Platform (UWP)
- Fluent Design System

Custom Dialogs in WinForms:
https://www.codeguru.com/columns/dotnet/custom-dialogs-in-winforms.html
Transparent Labels in WinForms:
https://weblog.west-wind.com/posts/2008/feb/07/transparent-labels-in-winforms
Unifying Web and Windows Form design and layout:
https://www.codeproject.com/Articles/2554/Part-I-Unifying-Web-and-Windows-Form-design-and-la
https://www.codeproject.com/Articles/2569/Part-II-Web-Window-Form-Unification-Synchronous-An
WinForms: Cần nắm vứng các khái niệm về Control, Component, Delegate, Event


DevExpress:
Custom controls:
https://10tec.com/articles/why-datagridview-slow.aspx
https://www.codeproject.com/Articles/16009/A-Much-Easier-to-Use-ListView
https://stackoverflow.com/questions/10226992/slow-performance-in-populating-datagridview-with-large-data
Security
https://www.red-gate.com/simple-talk/dotnet/windows-forms/controls-based-security-in-a-windows-forms-application/
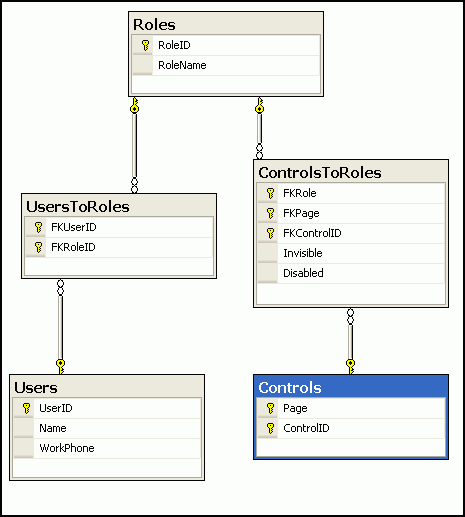
Menu
http://www.java2s.com/Code/CSharp/GUI-Windows-Form/DynamicMenu.htm
ListView
https://www.codeproject.com/Articles/16009/A-Much-Easier-to-Use-ListView
WinForm Sample
https://www.codeproject.com/script/Articles/ArticleVersion.aspx?aid=614673&av=898829
http://www.c-sharpcorner.com/UploadFile/c5c6e2/dynamic-menustrip-in-windows-forms/
Create Dynamic Menu In Windows Form C#
Dynamic Menu and Windows Form C#
https://www.codeproject.com/Articles/6074/Dynamic-MainMenu-formation-in-WinForm-Applicatio
https://www.codeproject.com/Articles/6043/Dynamic-MainMenu-formation-in-Winform-Application
https://www.codeproject.com/Tips/759515/Populate-MainMenu-Dynamically-in-VB-NET-Using-Recu
pkimmel@softconcepts.com
http://www.codeguru.com/csharp/csharp/cs_misc/designtechniques/article.php/c15661/Create-a-Dynamic-Menu-Using-C.htm
http://www.codeguru.com/csharp/csharp/cs_misc/designtechniques/article.php/c15571/Creating-a-Most-Recents-Menu-Item-with-the-MenuStrip.htm
https://www.codeproject.com/Articles/12204/Flat-style-menu-bar-and-popup-menu-control-for-Win
https://www.codeproject.com/Articles/22780/Super-Context-Menu-Strip
Video
https://www.youtube.com/watch?v=bkh1G6cbqPI
https://www.youtube.com/watch?v=lVH_UjJMOPo
Chia sẻ một vài kinh nghiệm lập trình WinForm
Trong quá trình làm việc với vị trí như một người phát triển sản phẩm, tôi có một số kinh nghiệm muốn chia sẽ cho những người mới làm quen như sau. Xin giới thiệu mình cũng khá rành về SQL Server, từ trước đến nay từng lập trình trên Fox và C#, kinh nghiệm triển khai phần mềm kế toán và SAP Business One từ năm 2006 đến nay, hiện vẫn viết Winform App bằng C# và SQL cho một công ty phần mềm tại TPHCM.
Với các control, hồi mới làm quen với C#, mình mê mẩn với bộ công cụ DevExpress, phải công nhận là công cụ của nó cực đẹp và dễ dùng. Sau này có kinh nghiệm hơn một chút, và đi làm thực tế, khách hàng và kể cả công ty mình khá tôn trọng bản quyền phần mềm (khá thôi nhé), do đó dần dần mình hình thành một quan điểm là hạn chế tối đa xài đồ chùa, đồ crack hết mức có thể. Từ đó mình nhận ra rằng DevExpress thì đẹp thật, nhưng tiền mua thì không có, mà crack thì lại phạm vào tinh thần tôn trọng bản quyền, từ đó mình tìm cách từ bỏ các bộ công cụ của bên thứ 3, phần vì mặc dù đẹp và dễ dùng nhưng về cơ bản là có nhiều thứ mình không cần xài đến, lại làm nặng chương trình, và đặc biệt là phải mua hoặc crack, về lâu về dài, khi người dùng Việt Nam bắt đầu có ý thức tôn trọng bản quyền cao hơn thì những thứ đó cần phải tính đến.
Tuy nhiên, bộ control có sẵn của .Net dù khá đầy đủ nhưng vẫn còn nhiều hạn chế, ở đây mình chia sẻ hai hạn chế cơ bản của hai control sau:
1) TreeView:
Theo quan điểm cá nhân của mình thì cái TreeView này có vẻ như Microsoft không đầu tư để viết cho nó đàng hoàng, trên bản .Net 3.5 mình muốn mỗi node có một icon là coi như bó tay (hoặc có thể mình chưa biết làm), các bản sau thì không biết thế nào. Và đặc biệt, nếu datasource của mình có nhiều hơn hai thuộc tính (hai cột trở lên đối với DataTable) thì cũng bó tay nốt, trong khi đó với TreeView của DevExpress thì vô cùng đơn giản, gán dataSoure, ParentColumn, Childrend column là xong, lại có cả Binding nữa.
Nhưng xài của DevExpress thì lại vi phạm vào những thứ như mình nói ở trên, với lại cả bộ DevExpress đồ sộ như vậy chẳng lẽ lại chỉ xài có mỗi một control TreeView, mà có muốn xài một TreeView đi nữa thì cũng phải add 3,4 file đi kèm rất nặng. Cho nên mình đã thay thế nó bằng TreeAdvance hoàn toàn miễn phí trên codeproject.com, các bạn search từ khóa này sẽ ra, chịu khó nghiên cứu cách sử dụng một ít nữa thì xài rất thoải mái, lại gọn nhẹ, có cả source cho các bạn nghiên cứu nến chịu khó. Chi tiết tham khảo tại: http://www.codeproject.com/Articles/14741/Advanced-TreeView-for-NET
Hình minh họa cho project mình đang làm:
2) DataGridView
Control này thì quá đủ cho chúng ta xài, tuy nhiên khi datasource có dữ liệu lớn, khoảng 10.000 đến 100.000 dòng trở lên thì control này build lại rất chậm, nhưng khi thay thế nó bằng ObjectListView thì nó build gần như là ngay lập tức, do đó, để view khối lượng dữ liệu lớn, bạn có thể sử dụng ObjectListView thay thế cho DataGridView. Theo mình đây là một trong những control miễn phí tuyệt vời nhất. Chi tiết mời bạn tham khảo tại: http://www.codeproject.com/Articles/16009/A-Much-Easier-to-Use-ListView
Một vấn đề nữa với DataGridView là có nhiều khi bạn muốn chọn giá trị từ một danh sách có sẵn, danh sách thì tùy, có loại danh sách đơn giản, nhỏ, thì có thể sử dụng column kiểu ComboBox là đủ, nhưng với danh sách dạng lớn thì làm thế nào, bạn muốn có một button bên trong Cell để chọn như DevExpress có thể làm? Điều này thì DataGridView không có, khi này bạn có thể thay đổi suy nghĩ một chút, không được vậy thì thêm vào một column bên cạnh, kiểu là button, với thuộc tính Width nhỏ thôi, vậy là người dùng chỉ cần click vào đó là có thể chọn danh sách được rồi, nó không đẹp bằng DevExpress nhưng cũng không tệ chút nào, thậm chí là khá đẹp. Như ví dụ sau
3) Report:
Có một số bạn có quan điểm rất sai lầm là coi nhẹ việc viết và thiết kế báo cáo, nhưng theo mình, đó là việc quan trọng, nếu không muốn nói là gần như quan trọng nhất, bạn nhập liệu và lưu trữ, nhưng nếu không trình bày được dữ liệu đó ra theo những định dạng như nhà quản lý yêu cầu thì phần mềm của bạn chỉ mới đạt được 2/3 chương trình đó là thu thập và lưu trữ dữ liệu, chứ chưa thể đóng vai trò phân tích dữ liệu được. Do đó, một phần mềm được coi là mạnh không chỉ tiện dụng ở việc thu lập và lưu trữ, mà còn phải xuất dữ liêu đó ra theo các báo cáo với các tiêu chí và mục đích khác nhau của nhà quản lý. Do đó, việc lựa chọn công cụ để thiết kế báo cáo cũng cực kỳ quan trọng không kém.
Theo mình thấy, trong .Net hiện nay có hai control cho thiết kế report là Crystal Report và Report Control. Ý kiến của mình là nên sử dụng Report Control của Microsoft hơn. Report Control theo mình được biết thì ra đời sau Crystal Report, và Crystal Report cũng đã không còn là của Microsoft nữa mà được bán lại cho SAP từ lâu, Microsoft tập trung phát triển Reporting Service để thay thế cho Crystal Report, và Report Control là phiên bản rút gọn của Reporting Service. Bản này dễ sử dụng hơn rất nhiều. Mình từng thiết kế report trên Fox, Access, Active Report, Crystal Report, nhưng mình chưa thấy cái nào có thể làm tốt như Report Control trên .net cả. Với hệ thống phần mềm lớn với số lượng hàng trăm, hàng nghìn báo cáo thì control này sẽ tiết kiệm được lượng lớn thời gian cho các bạn làm được nhiều việc khác.
Bên cạnh đó, do các ứng dụng lớn có rất nhiều báo cáo, nếu bạn đang không dùng Reporting Service của MS thì bạn cũng nên tạo một cơ chế để tạo được tham số động để chạy các store lấy dữ liệu, vì thông thường các báo cáo cũng rất thường xuyên thay đổi, thêm bớt tham số… Điều này sẽ tiết kiệm được rất nhiều thời gian và giảm thiểu sự nhàm chán.
4) Connection
Việc kết nối từ Client đến SQL Server có thể theo cơ chế xác thực của Window hoặc của SQL, của Window thì bảo mật hơn, nhưng đồng nghĩa với việc khi triển khai bạn sẽ gặp khó khăn hơn do các máy phải đảm bảo kết nối thông suốt được với nhau trong mạng nội bộ hoặc thông qua VPN. Window xác thực cũng tránh cho việc những máy tính không nằm trong mạng của bạn không thể động chạm đến dữ liệu. Trường hợp không có VPN hoặc khó khăn trong việc triển khai cơ chế này, bắt buộc bạn phải NATing router, mở port SQL 1433 thì có thể tham khảo ở đây. Nếu bạn không muốn NAT và mở port SQL, tốt hơn là sử dụng một WCF service được host trên IIS và mọi hoạt động ở Client đều được kết nối thông qua Service này….
5) Menu
Với chương trình lớn, việc thay đổi, cập nhật menu diễn ra thường xuyên. Để làm nhanh hơn, bạn nên tạo ra một cơ chế có thể tạo menu động, toàn bộ thông tin của Menu sẽ được lưu trữ dưới database, mỗi lần thêm, bớt, sửa đổi bạn chỉ cần cập nhật nó thông qua giao diện là được, kinh nghiệm của tôi là làm điều này sẽ tiết kiệm rất nhiều thời gian.